Simple Bézier Curves in Matlab
I’ve always been curious about how [Bézier] cubic splines are generated and I how I can use them in various projects (game development probably being the most immediately obvious). If you don’t know what I’m talking about, Wikipedia has a decent if somewhat tedious description. After digging through the math, I came up with these results which I’ll share for the simplicity of it all (they really are simpler than I ever though). I found most sources started at the beginning and gave huge mathematical backgrounds, which although are nice, are typically just not what I was looking for. What I was looking for (and what is presented here) is just the end result—given a set of input weights, how do I calculate the actual spline?
First things first, this is the kind of spline I’m talking about:
Bézier splines are parametric curves, meaning instead of a function like:
you instead get functions like:
Where . This isn’t a big deal though, and in fact makes our lives even easier—we can just create a list of points in between 0 and 1 and get the corresponding x, y, [and z] coordinates that belong there:
t = linspace(0, 1);
The next thing we need to know is that and are just polynomials, defined as:
Where are just coefficients. These coefficients can be calculated as such:
and:
Or, if you’re not big into matrices and vectors:
Note that the equations are essentially the same for each dimension, so would follow the exact same format (note how the coefficients are calculated the exact same way as the ones).
In order to construct this in Matlab, we could do something like the following:
x = [0; 1; -1; 0];
y = [0; 1; 2; 3];
Such that we get points like:
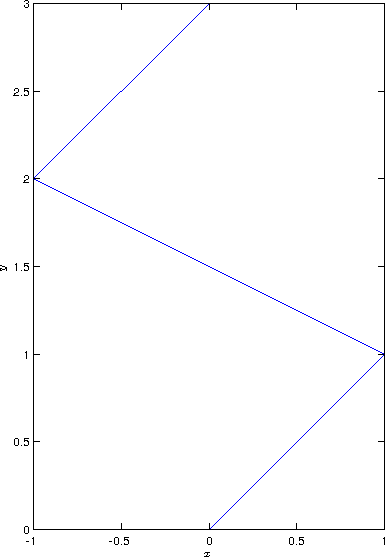
We can then calculate coefficients as such:
C = [-1, 3, -3, 1; 3, -6, 3, 0; -3, 3, 0, 0; 1, 0, 0, 0];
Cx = C * x; % Cx = [6; -9; 3; 0]
Cy = C * y; % Cy = [0; 0; 3; 0]
Finally, to create the parametric curve we can combine everything:
sx = polyval(Cx, t);
sy = polyval(Cy, t);
plot(sx, sy);
So that we get something like:
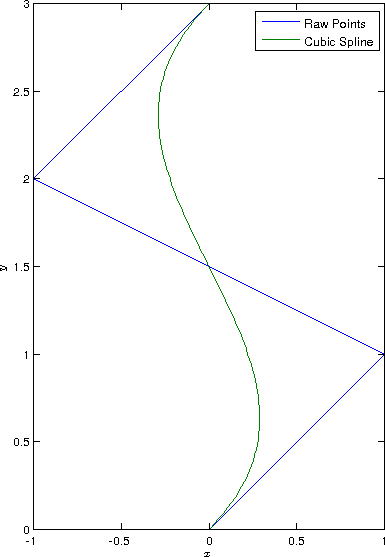
Note that the “resolution” of this is entirely dependent on the number of points that exist in t
. By default, in linspace
, this will be 100, but we can easily drop that down:
t = linspace(0, 1, 10);
sx = polyval(Cx, t);
sy = polyval(Cy, t);
plot(x, y, sx, sy);
Which results in:
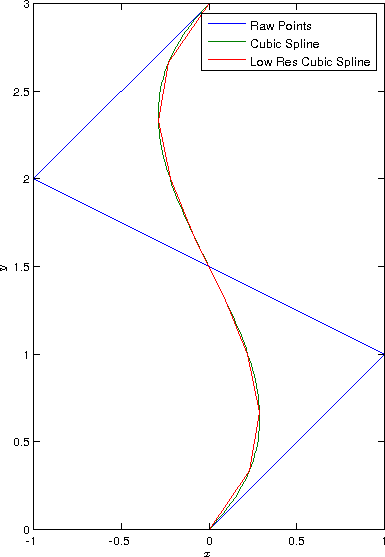
And we’re done! That was a lot easier than I was expecting!
Note that this formulation is just for one segment that would typically be part of a much longer line composed of multiple segments. If we wanted to connect two segments together, in order to make the connection smooth all we have to do is ensure the slope of these curves are equal. The easiest way to do this would most likely be to calculate in the second segment using and from the first segment such that:
(Use the same formulation for the dimension).
I’ll probably post more things later, such as how to calculate the length of each spline segment so that we can do things like determine appropriate resolutions and calculate total lengths of lines etc, but hopefully this will get you off to a start right now!